Python : MQTT Console publisher and consumer
|MQTT is a popular pub/sub protocol in IoT space. Its lightweight and has client libraries for several IoT platforms. We have already seen how to use MQTT on nodemcu and ESP32. Its convenient to have a console publisher for testing and debugging purpose. In this post I will present a simple python program that will read standard input and publishes to the MQTT topic.
Environment setup
Python setup
if you don’t have a working python setup, first install python. Then install we need to install paho mqtt client.
pip install paho-mqtt
MQTT Broker
mosquitto is a popular open source MQTT broker, which can be installed on local machine. mosquitto broker can also be installed on raspberry pi. There are several cloud providers also. For this post I will a open broker provided by eclipse foundation.
eclipse open broker details
host name : mqtt.eclipseprojects.io
port : 1883
Python console publisher
Console publisher is a simple python program. Publish topic name need to provided as a command line argument. Program will publish every line that is available from the standard input. It will strip the newline before publishing the message. Full documentation for paho client is available here.
import paho.mqtt.client as mqtt import sys mqttc = None def on_connect(client, userdata, flags, rc, properties): print("Connected with result code "+str(rc)) def listen_std_in(topic): print("sending messages to ",topic) for line in sys.stdin: if 'exit' == line.rstrip(): break else: mqttc.publish(topic,line.rstrip()) if __name__=="__main__": mqttc= mqtt.Client(mqtt.CallbackAPIVersion.VERSION2, 'ic-mqtt-console-publisher') mqttc.connect("mqtt.eclipseprojects.io", 1883, 60) mqttc.on_connect=on_connect mqttc.loop_start() # non blocking call listen_std_in(sys.argv[1]) mqttc.loop_stop() print("DONE.")
Example usage
Copy above program into a file and execute the program as the following image shows. Once program starts and text entered in the console will be published to the topic.
python <file name> <topic name>

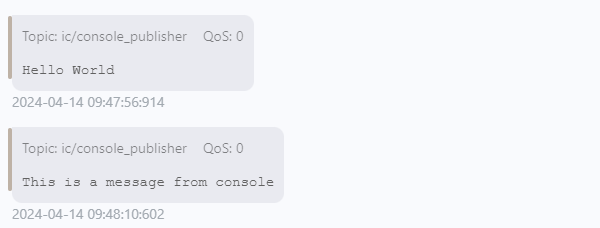
Python console consumer
Similar to publisher, we can also write a simple message consumer. The following python program will subscribe to the provided topics and prints the received messages on console. Multiple topics can be provided separated by comma.
import paho.mqtt.client as mqtt import sys mqttc = None topics = None def on_connect(client, userdata, flags, rc, properties): print("Connected with result code "+str(rc)) if not rc.is_failure: listen_mqtt_topics(client,topics) def on_message(client, userdata, message): print("{} {}".format(message.topic, message.payload)) def listen_mqtt_topics(client,topics): print("topics ",topics) for topic in topics: client.subscribe(topic) if __name__=="__main__": mqttc= mqtt.Client(mqtt.CallbackAPIVersion.VERSION2, 'ic-mqtt-console-consumer') mqttc.connect("mqtt.eclipseprojects.io", 1883, 60) topics = sys.argv[1].rstrip().split(",") mqttc.on_connect=on_connect mqttc.on_message = on_message mqttc.loop_start() # non blocking call input("Press enter to terminate") mqttc.loop_stop() print("DONE.")