Arduino ESP32 : How to get time from NTP Server
|Networked digital devices can get time by sending a NTP request to any one of the NTP servers available on the internet. This is very use full service for devices that don’t have an on board time keeping chip such as DS1307. With out these time keeping chips, computers/microcontrollers don’t have a way to keep time when they are rebooted. Using NTP, these devices can get current time on startup or periodically. Then use internal clock sources to keep it in sync.
There are several NTP servers available such time.google.com, pool.ntp.org, time.nist.gov etc. I have already discussed about a simple client for NTP. ESP32 already provides a full featured sntp library, so we don’t have to use the simple client. Arduino ESP32 also provides a time wrapper for easier use of the library.
Objective
We would like to print current date and time on serial console periodically. This objective can be extend to show the data and time on a LCD or 7-segment display. In this post we will only print the data and time on the serial console.
What you need
Any ESP32 Development board
PC to program ESP32
Solution approach
Solution is straight forward. We connect the ESP32 to a access point that has internet connection, then fire a NTP request and capture the timestamp from response. most of this will be handled by the library, user code just need to set the time zone information and access point information.
Code
We set the time zone and connect to a WiFi access point in setup block. I am using pool.ntp.org NTP provided, other NTP servers will also work.
struct tm { int tm_sec; int tm_min; int tm_hour; int tm_mday; int tm_mon; int tm_year; int tm_wday; int tm_yday; int tm_isdst; }
getLocalTime method will set the time and details in the tm struct passed to it. The method will return false if the timestamp information is not available. We can access individual fields from struct and prepare a string using sprintf method. I listed all the available fields in the tm struct above. For example to print hours and minutes we can write a format string as follows
sprintf(printBuffer, "%d:%d", timeinfo.tm_hour, timeinfo.tm_min); Serial.println(printBuffer);
There is also a standard method available for formating the strftime
There is a standard method strftime available for formatting time struct. There are lot of formatting specifier available, please check the documentation of strftime more info. The method takes the character buffer, max length, formatting string and the tm struct. Writes the formatted string into provided character buffer. The written string is null terminated, so we can directly pass it to the println method.
#include <WiFi.h> #include "time.h" const char* ssid = "***"; const char* password = "***"; const char* ntpServer1 = "pool.ntp.org"; const char* time_zone = "IST-5:30"; void printLocalTime() { struct tm timeinfo; if(!getLocalTime(&timeinfo)){ Serial.println("No time available (yet)"); return; } char printBuffer[100]; sprintf(printBuffer, "Year %d, Month %d, Day %d, Hour %d, Min %d, Sec %d", timeinfo.tm_year+1900, timeinfo.tm_mon+1, timeinfo.tm_mday, timeinfo.tm_hour, timeinfo.tm_min, timeinfo.tm_sec); Serial.println(printBuffer); strftime(printBuffer, 100, "%A, %B %d %Y %H:%M:%S", &timeinfo); Serial.println(printBuffer); } void setup() { Serial.begin(115200); configTzTime(time_zone, ntpServer1); //connect to WiFi Serial.printf("Connecting to access point"); WiFi.begin(ssid, password); while (WiFi.status() != WL_CONNECTED) { delay(500); Serial.print("."); } Serial.println(" CONNECTED"); } void loop() { delay(5000); printLocalTime(); }
Testing
Update the ssid, password and set the time_zone string. You can get list of available timezone configs from here. Connect ESP32 to PC and select the COM port in Arduino, then upload the code. If you open the serial console after uploading code, you should see the time being printed in it every 5 seconds.
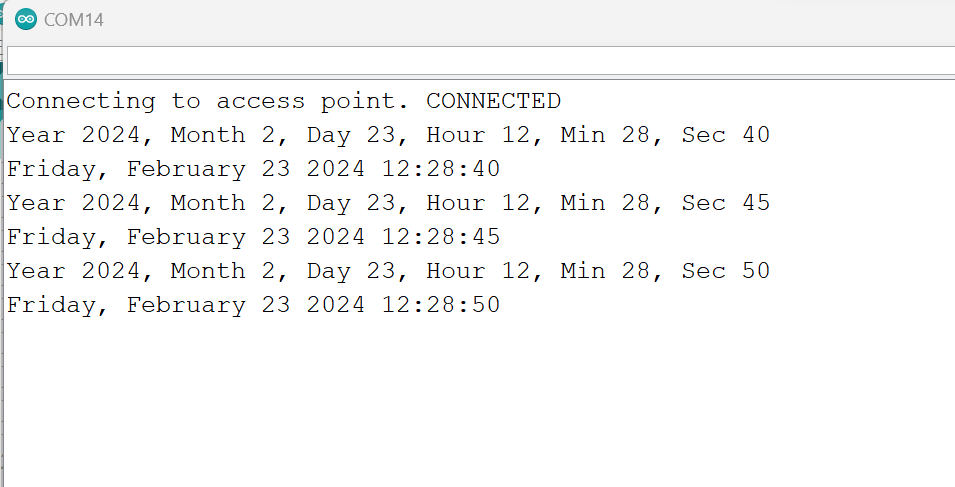