HOW TO MAKE ESP32 AS HTTP WEBSERVER USING Arduino ?
|Data centers and racks full of servers with multi-core processors comes to mind when we think of web servers. For small webpages, we don’t need all these big servers, little microcontrollers can serve them (due to resource constraints, these controllers can serve only hand full of concurrent clients, where as the machines in data centers can serve upto few thousand concurrent users). In this post, we will see how to serve a web page from ESP32. The server running on ESP32 is not exposed to internet, so only the clients on the same network as that of the ESP32 can access the webpage.
What you need
- Any ESP32 based development board
- PC to program ESP32 and test the web page
you can add ESP32 to arduino IDE by following this post.
Objective
We want to be able to serve a webpage from ESP32. We can see this web page in a browser by sending a request to the web server running on ESP32.
Approach
We will use the arduino WebServer library and register a GET endpoint that will return the “Hello!”. We can also pass a URL parameter called “person”, then response will include the value of the parameter. The library can handle only one client at a time, that will not be problem for our use case.
Code
#include <WiFi.h>
#include <WiFiClient.h>
#include <WebServer.h>
const char* ssid = "***"; // wifi access point name
const char* password = "***";
WebServer server(80);
void handleHello() {
if(server.hasArg("person")){
server.send(200, "text/plain", "hello "+server.arg("person")+"!");
} else {
server.send(200, "text/plain", "hello!");
}
}
void setup(void) {
Serial.begin(115200);
WiFi.mode(WIFI_STA);
WiFi.begin(ssid, password);
Serial.println("");
while (WiFi.status() != WL_CONNECTED) {
delay(500);
Serial.print(".");
}
Serial.println("");
Serial.print("Connected to ");
Serial.println(ssid);
Serial.print("IP address: ");
Serial.println(WiFi.localIP());
server.on("/hello", handleHello);
server.begin();
Serial.println("HTTP server started");
}
void loop(void) {
server.handleClient();
delay(2);//allow the cpu to switch to other tasks
}
A web server can have server different resources (web pages) on different URLs, each URLs will have handler on the server. The WebServer library provides the on method, using which we can register handlers (methods), that handles the request came for the endpoint. handleHello method will be called by the library when a request comes for <host>/hello URL.
server.on("hello",handleHello)
The handle method will check if there is parameter/argument with the name “person”. if the argument is present it will say hello to that person otherwise just sends simple “Hello!” message.
void handleHello() {
if(server.hasArg("person")){
server.send(200, "text/plain", "hello "+server.arg("name")+"!");
} else {
server.send(200, "text/plain", "hello!");
}
}
How to test the WebServer
Upload the above code to ESP32 and open the serial console. Make sure to change the WiFi access point details in the code before uploading. Once the ESP32 is connected to the WiFi, it will print the IP on which ESP32 started the webserver.
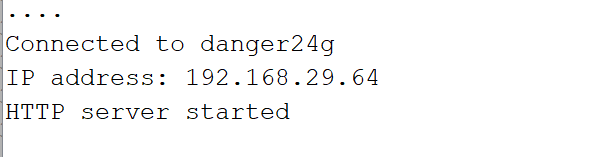
Open a web browser tab and enter the following URL (replace the IP in the URL with your ESP32 IP)
http://192.168.29.64/hello
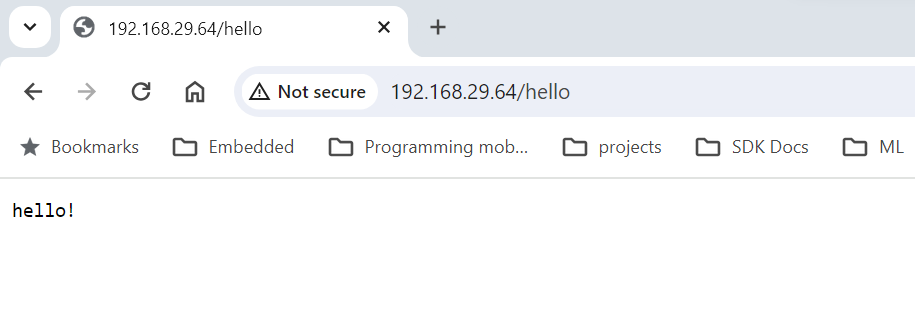
If we add the person URL parameter, then we will see the value of the parameter added in the response.
http://192.168.29.64/hello?person=Alexa
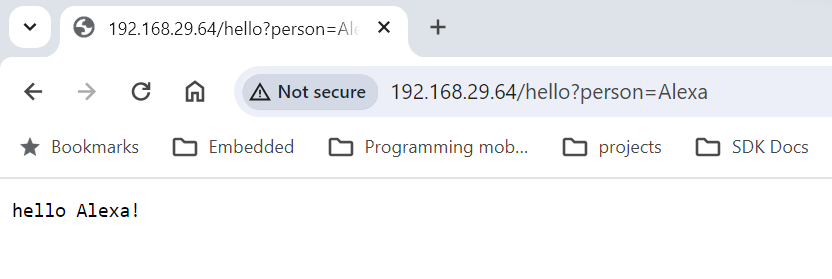