Arduino : 4 digit 7 segment clock with ESP32 and TM1637
|Digital clocks are affordable and ubiquitous. In this post, we will build a digital clock using ESP32 and TM1637 on Arduino platform. There are several TM1637 based 4 digit 7-segment displays available in the market. TM1637 is an inexpensive LED driver come keypad scanner. Please see INTERFACING CONTROLLERS WITH TM1637 if you want understand how this chip works.
Objective
We would like build a digital clock using ESP32 and TM1637 based 4 digit 7-segment display. The digital clock will show hours and minutes in 24 hours format.
What you need
- ESP32 development board
- TM1637 based 7 segment display
- Jumpers for connecting ESP32 with TM1637
- PC to load the program ESP32
Solution approach
Typically digital clocks will have an RTC chip such as DS1307 and coin cell battery to maintain time in case of a power cycle. ESP32 has WiFi, So we can use its network capability to fetch the time from NTP servers on startup. Several NTP server on internet provide free time service. See building a NTP client for Arduino ESP32 post to understand how NTP works. In that post I wrote a simple NTP client to demonstrate inner workings of NTP, for digital clock we don’t need to write our own client, ESP32 already provides NTP library. See Getting time from NTP server using Arduino ESP32 to understand how to use time.h library provided by arduino-esp32 platform.
Connections
ESP32 | TM1637 Board |
3V3 | VCC |
GND | GND |
22 | CLK |
19 | DIO |
Code for ESP32 based Digital Clock
In the setup block we initialize the timezone and setup the 7 segment display. After that we are connecting to the configured WiFi. We are not reading any data from the TM1637 chip, so we are putting both Clock pin and Data pin in OUTPUT mode (If there is a need to read from TM1637, we need to put the Data pin in INPUT mode).
TM1637 supports 8 levels of brightness, we are initializing it with maximum brightness by sending 0x8F command.
// switch on the display uint8_t displayControlCmd[1]={0x8F};// display on with full brightness sendByteStream(displayControlCmd,1);
In the loop block we are refreshing the display every second by calling the updateTime method. In the updateTime method, we load the time into timeinfo tm struct by passing its reference to getLocalTime method. We are converting the hours and minutes to 4 character string using sprintf method. The %02d format specifier will prefix 0 if the given number is single digit. The sprintf method will return number of characters written to the buffer, we are capturing it in the strLen variable.
int strLen = sprintf(printBuffer, "%02d%02d", timeinfo.tm_hour, timeinfo.tm_min);
We can’t directly write these characters to TM1637 chip, we have to encode them to 7 segment LED pattern. Each digit has a specific 7 segment pattern. We have placed these hex codes in PATTERNS array. The index of the pattern matches with digit it encodes. For example number of 5 pattern will be available at index 5 and can be access using PATTERNS[5]. We converting the ascii characters 0-9 to the index by subtracting 48, 48 is the ascii value of the character ‘0’. Some 4-digit 7 segment displays will have a colon (:) at the center. We need to set the first bit of the pattern to enable the colon. To make it blink, we show it only every alternate second.
uint8_t tokenBuffer[NUM_DIGITS+1] = {0}; tokenBuffer[0]= {0xC0}; // first byte will be the register address uint8_t showColon = timeinfo.tm_sec % 2; for(int i=0;i<strLen && i<NUM_DIGITS ;i++){ uint8_t digit = ((uint8_t)printBuffer[i])-48; tokenBuffer[i+1] = PATTERNS[digit]; if(showColon == 1){ tokenBuffer[i+1] = 0x80 | tokenBuffer[i+1]; } }
The tokenBuffer can directly be streamed to TM1637. So we are calling sendByteStream method with tokenBuffer and length. The first byte of the tokenBuffer has the address of the display register that controls the first 7-segment display.
Testing ESP32 Digital Clock
Make the connections as per the table above. Make sure to set the ssid,password and timezone before uploading the code to ESP32. Supported timezone list is available here. Open the serial console after uploading the program, you should see time being printed on serial console every second. If the 7-segment display is connected, it will also get refreshed every second.
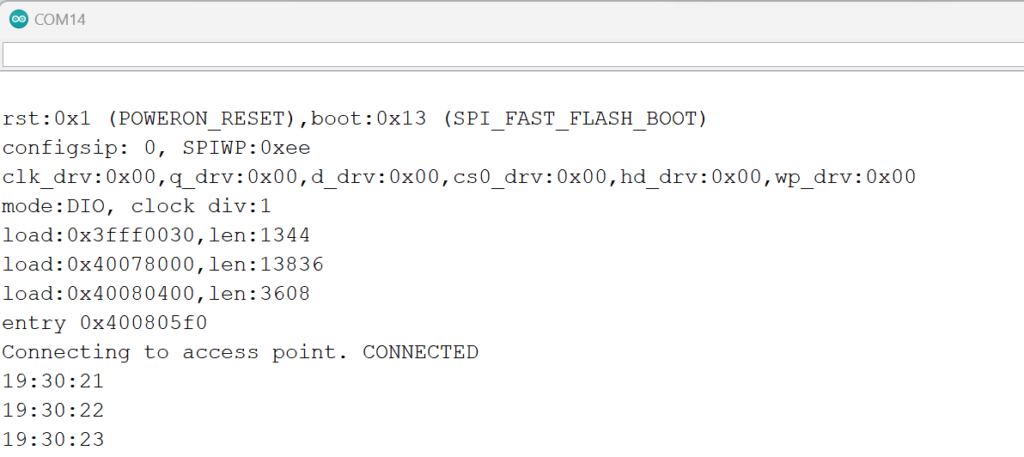