Arduino: ESP32 Hardware Serial2 Example
|ESP32 has three hardware UART controllers (Serial ports) , UART0, UART1 and UART2. All three UART controllers are independent and fully featured (configurable parity bit, baud rate etc.). Like other peripherals on ESP32, these UART controllers can be mapped to IO ports on the chip.
In this post we will see how to use Serial2 (UART2) from arduino code. To demonstrate its working we will write a loopback program.
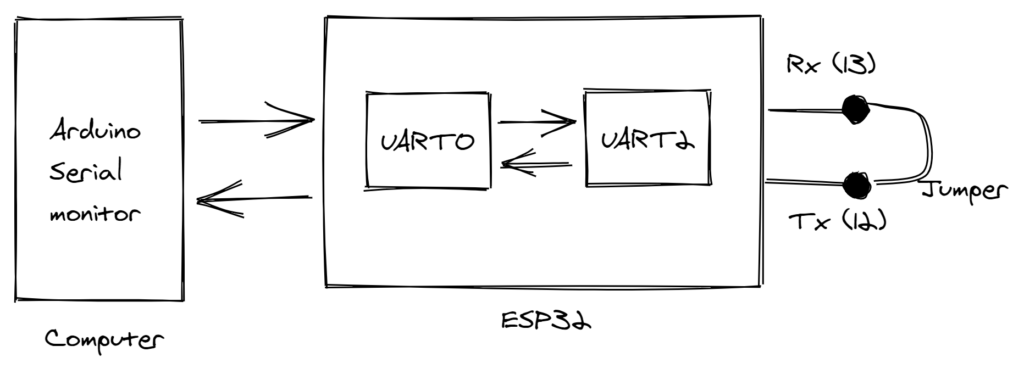
The working is simple, we write something in arduino serial console and program will echo it. The serial console is connected to Serial (UART0), which is also used for loading arduino code to ESP32. The program will be monitoring UART0, and if it sees any data on UART0 it will write that data to UART2. Similarly any data received on UART2 will be written to UART0 (which we can see in arduino serial console). For the loopback to work we need to connect the UART2 Rx, Tx pins together. Connect UART2 Rx, Tx pins with a jumper (For this case connect 12, 13 pins together with a jumper wire).
#define RXD2 13 #define TXD2 12 void setup() { Serial.begin(115200); Serial2.begin(115200, SERIAL_8N1, RXD2, TXD2); delay(1000); Serial.println("Loopback program started"); } void loop() { if(Serial.available()){ Serial.write("-"); Serial2.write(Serial.read()); } if(Serial2.available()){ Serial.write("."); Serial.write(Serial2.read()); } }
If you load the script, you should see something like below in your serial console (of course you need to type in LoopBack Program and click on send button).

Very clear information about serial communication.
Thanks & Regards.