Java reference types
|In general we only encounter Strong references in Java program. But there are few other types of references as well. In this post we will see different types of references available in Java
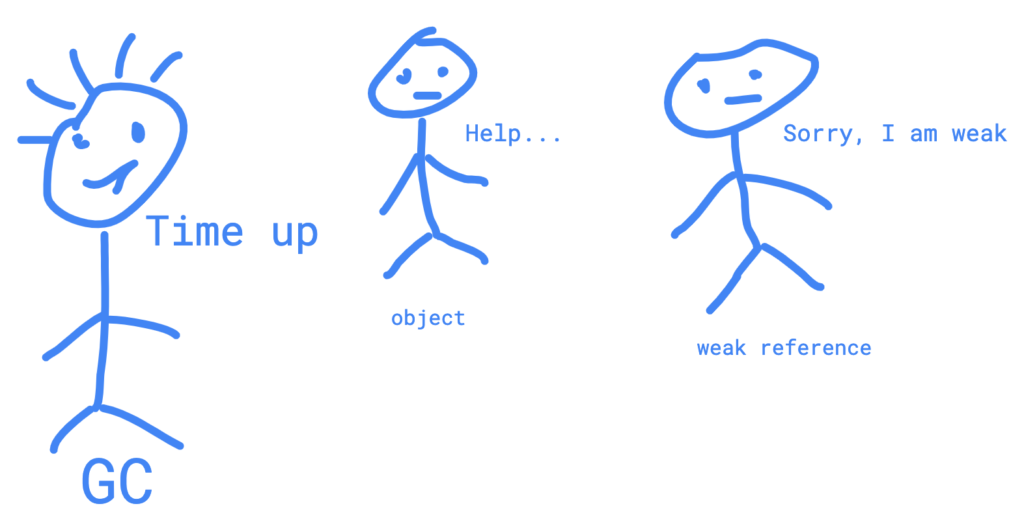
Different reference types available in JVM
- Strong reference
- Soft reference
- Weak reference
- Phantom reference
JVM GC treats these different references in different ways.
Strong Reference
When we create an object and capture the reference in a variable, we have a strong reference to that object.
Employee e = new Employee()
As long as we have a strong reference to an object, It will remain in memory (It will not be garbage collected).
Weak Reference
The objects that are only reachable by a weak reference will be garbage collected in GC cycle. Weak reference doesn’t prevent an object from being garbage collected.
WeakReference<Employee> wrEmployee = new WeakReference<>(new Employee());
We can get the employee object from weak reference using get() method. This method might return null as well, so we always have to check for the null
Employee employee = wrEmployee.get()
Soft Reference
Soft references are similar to weak references except that with soft references GC will remove the object from memory only when there is memory pressure.
Soft references lets us keep an object in memory as long as possible. GC will remove an object from memory when it has to reclaim some memory.
Phantom Reference
When GC determines that an object is only reachable through a phantom reference, it enqueues the phantom object with registered ReferenceQueue. Then user code can run some cleanup code and clear the reference from the phantom object. GC will not automatically clear the reference object from phantom like it does for soft and weak reference objects, it has to be cleared manually or the phantom object itself need to be garbage collected. The phantom get method always returns null to avoid resurrecting the referred object after it is enqueued.