WSL: Working with COM ports on Windows/Linux
|With WSL we can access windows COM ports from Linux programs. In this post we will see how to access COM port from Python and NodeJS programs.
Refer this post if you haven’t installed WSL on your windows machine. There is a simple mapping between windows COM port to Linux tty interface. COM<N> is mapped to ttyS<N>, for example for COM5 the device name in WSL would be /dev/ttyS5.
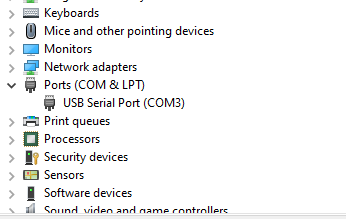
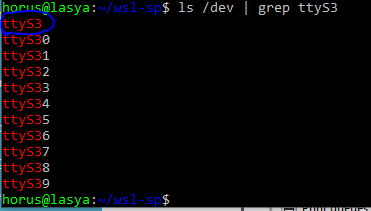
Accessing COM port from Python
we will use pySerial to connect with serial port. Any existing python program should work if we just change the serial port name to `/dev/ttyS<N>`, where N is the COM port number. You can get the COM port number from device manager window.
mkdir wsl-python-sp cd wsl-python-sp pip install pyserial
copy following code into hello.py
import serial ser = serial.Serial('/dev/ttyS3') # open serial port print(ser.name) # check which port was really used ser.write(b'serail port on wsl \n') # write a string s = ser.readline() print(s) ser.close()
If your serial device is in loop-back mode (its writes back what it receives), you will see the following message on console
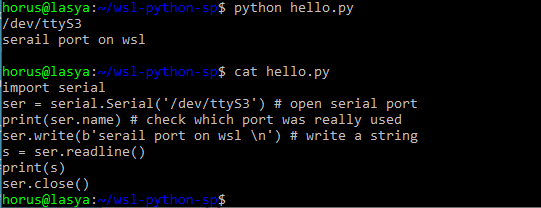
Accessing COM port from NodeJs
for NodeJs we can use serialport package for serial port access. Open your WSL console and run following cmds.
mkdir wsl-sp cd wsl-sp npm init npm install serialport touch index.js
open index.js and copy following contents (Change the serial port name appropriately).
const SerialPort = require('serialport') const Readline = require('@serialport/parser-readline') const port = new SerialPort('/dev/ttyS3', { baudRate: 9600}) const parser = new Readline() port.pipe(parser) parser.on('data', line => console.log(`> ${line}`)) port.write('serailport on wsl\n')
now run the script
node index.js
If your serial device is in loop-back mode (its writes back what it receives), you will see the following message on console
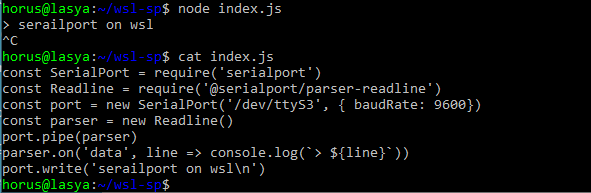