Arduino: Interfacing Arduino UNO with ESP32
|We have already seen how to connect arduino uno to NodeMCU (ESP8266) here . In this blog we connect Arduino UNO with ESP32. When connecting UNO with nodemcu we used Lua to program the ESp8266 device, here we will use arduino IDE to program both UNO and ESP32.
Just like in the previous post, UNO has some JSON data that its want to publish to a MQTT broker (actually it doesn’t matter what data UNO is sending,ESP will just consume data until it sees newline char,once newline char is received it will send the received data to the MQTT broker). Since UNO can’t do that alone, it will send that on a SoftSerial line to ESP32. We will program ESP32 to send every new line appeared on serial line to MQTT broker on specified channel.
Environment requirements:
- you need to have UNO and ESP32
- you would also need a 5v to 3v3 level converter to convert the UART signal levels
- setup your Arduino IDE to program ESP32
- Arduino libraries : PubSubClient (if you haven’t already installed it , you can install it from Sketch->Include library->Manage Libraries)
- mqtt broker , if you don’t have one you can use eclipse Paho broker for experimentation (host: mqtt.eclipse.org , port : 1883, it is a free and open broker)
Solution Approach:
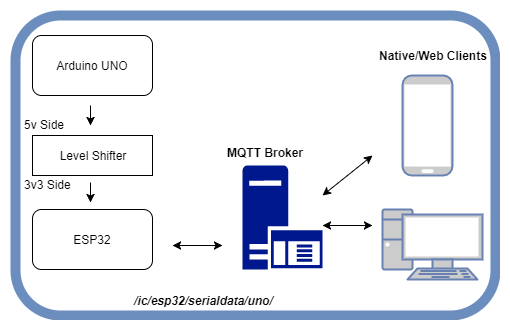
We will push the data received on serial line on “/ic/esp32/serialdata/uno/” channel/topic. You can view the data sent by the UNO on any MQTT client by subscribing to the topic “/ic/esp32/serialdata/uno/“, I also embedded MQTT client at the end of this blog, scroll down to see it. Put your Broker details (if you don’t have a broker you can use the defaults and click on connect, when ever UNO sends a new message it should appear here)
First we will write program Arduino UNO to generate periodically then we will move to ESP32 code
Arduino UNO code:
Nothing fancy on arduino side, you take sample form ADC and send it over softserial . load the following code to arduino uno board (don’t forget to change the board and COM port).
ESP32 Code:
On ESP32, we need to do the following
- connect to WiFi (Access point)
- connect to MQTT broker
- send every new line received on serial line to MQTT broker
Change the access point details and MQTT broker (if you have a private instance otherwise use the defaults) Load the following code to ESP32 (don’t forget to change the Board and COM port settings in the IDE)
How To Test:
Connect UNO to system and open serial console. You should see something like the following

Connect ESP32 and change the COM port appropriately. Open serial console, it should print IP after connecting to Access point
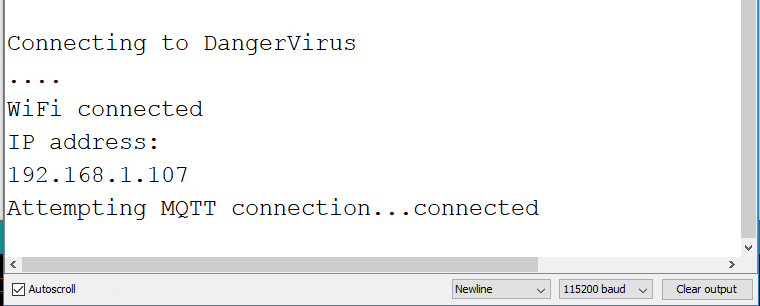
If both boards are working as expected, we can connect them together now. Connect Arduino UNO pin 3 to the Rx pin of ESP32 through Level Shifter.

Click on Connect button below and power up both boards,you should see the messages below or in your MQTT Clinet.
——————————————————————–
——————————————————————–
Very interesting project. I am asking you a suggestion how to modify my present project by making use of your hints. My project acquire dat from several sensors and store them in a SD card of an Arduino data logger shield (Adafruit like). Data are written as matrix rows separated by tab character and terminated by a return character. When I open the Arduino monitor the rows are regularly written each time they are generated.
The ESP 32 is already ready by a server dedicated to control some switches bui I can change it.
My question is:
– Can I connect RX an TX of Arduino directly to TXD0 and RXD0 providing the level shifter according to your scheme? Is it right excluding the USB connection to PC adding to Arduino program the line “SoftwareSerial sw(0, 1);”?
Many thanks
Hi , Yes You can directly connect ESP32 to Arduino Hardware UART with level shifter. In this scenario you can’t use Arduino USB serial. If you wan’t use arduino serial monitor, you need to use software serial on arduino to connect with ESP32
Thank you for your prompt reply. I am not interested to use Arduino serial monitor. I am very interested to read on ESP32 the data acquired by Arduino/Data Logger Shield. Because I am not familiar with MQTT, I ask you how your ESP32 code changes if MQTT is not adopted.
Thank you for your cooperation
Regards
Dan
Hi,
I have recently bought ESP32 and already having arduino IDE with me. Can I connect ESP32 directly with to Arduino UNO without logic level converter? Kindly reply me asap.
You should use level converter
Hello sir,
I am sending data to cloudmqtt.com server by using GSM at commands but error is showing on terminal. Could you please tell me how to send data to server using GSM.
Your any help is appreciable.
Thanks and Regards
palak
Hi,Thanks for a nice tutorial.Please updat iot.eclipse.org to mqtt.eclipse.org.
Syam
Thank you
Hi, when I connect my esp32 to the LV and GND of the level converter the LED doesn’t light up which indicated that the esp32 is not powering up although I connected the HV and GND pins in the arduino. Have any ideas why it doesn’t work?
You need to power esp32 as well (using another power source)
Thanks, made my day.
Simple and excellent explained.
Fabulous tutorial!!! By the way: Are you the author of any books? Your didactics is fantastic!
I’m new to arduino coding. May i know how to write the coding if i want publish the pH and EC data from arduino uno to esp32 and lastly to nodered mqtt?
Hello,
i am able to get individual message in serial monitor as the picture attached.
But where do i need to see the data send by wifi….
In the box you attached at last. by default
Host is mqtt.eclipse.org
websocket port is 443
i enter username as mqtt username
and password as mqtt password
and click connect
it just shows
Message from ESP32
—————-
Weather my modules are power On or OFF.
Just let me know where to see the data after whole connection and communication is established.
In serial monitor …. connected shows…
nothing after that.
hello,
I repeated every step.
got both the message indivdually as the pic attached.
But where do i see the wifi transmitted data after the uno and ESP are connected together.
In the last section u attached …
its host default is mqtt.eclipse.org
websocket port is 443
i entered username as mqtt username
and password as mqtt password
so when i click connect
it just shows
Message from ESP32
——————————-
and nothing else.
even in serial monitor after connected,… nothing shows.
Just help me where and how do i see my wifi transmitted data.
Hi,
Everything is okey. but i am unable to see the data transfer from wifi.
Where do i need to see the message.
as after clicking connect it just shows
Message
————
you take sample form ADC and send it over softseria
…. how do I do this please ?