interfacing arduino uno with nodemcu
|NodeMCU is great for connecting cloud and arduino is great at talking with different sensors. nodemcu has only one analog pin. In this blog we will see how to connect arduino to nodemcu and post data to a mqtt broker. Arduino will take temperature readings and send the readings to nodemcu over serial connection. Nodemcu will send a mqtt message for every reading it receives. If you have just one sensor to monitor you can directly use the analog input available on nodemcu, see this blog on how to use the analog pin of nodemcu.
On Arduino side, we will take sample periodically and send a JSON message over softserial to nodemcu. Please note that Arduino uno works on 5v and nodemcu works 3v3 level. So you should use a level shifter to connect arduino soft serial pins to the nodemcu uart port (It also works without level converter , but it is not recomended).
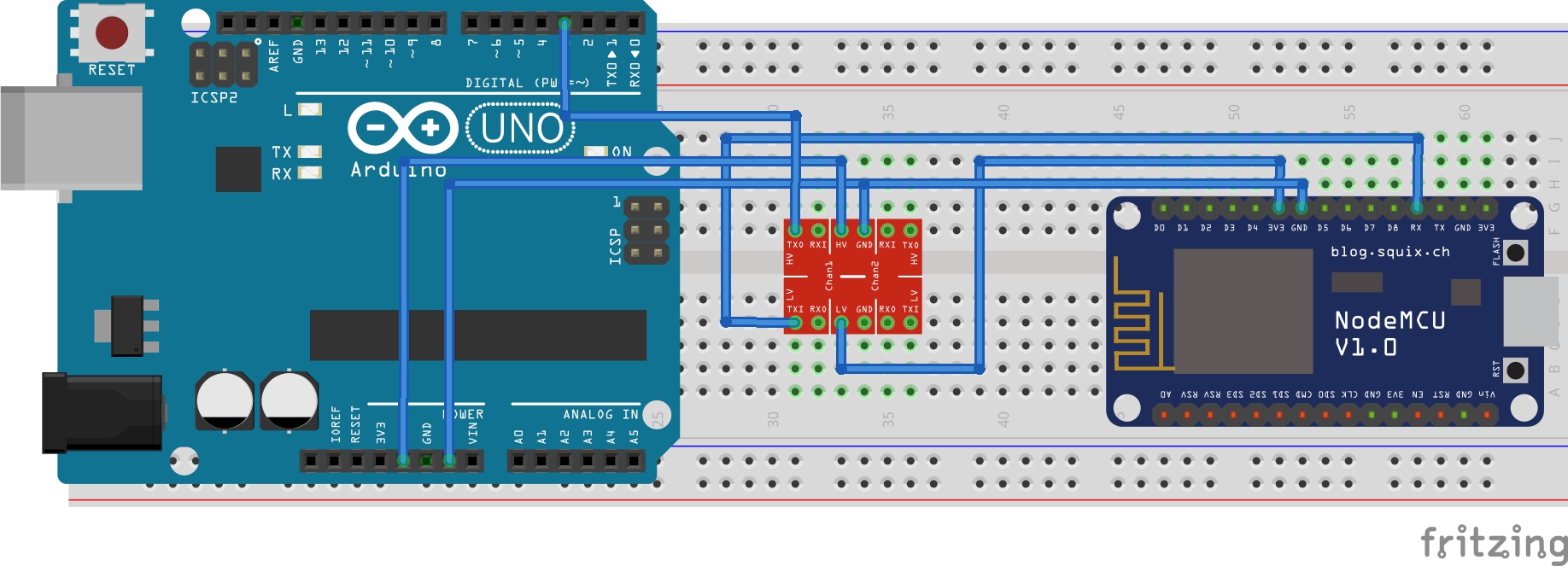
On nodemcu side we need to receive the messages sent by arduino over serial. Arduino will send the messages in JSON format. We will not be doing any processing on nodemcu (It is possible to process the messages before sending to cloud, for example you can compare the present reading with the previous published messages and send the message only if the reading is different) . You need to have working MQTT broker account. You can follow this blog to create and test mqtt account.
Arduino Code :
Nothing fancy on arduino side, you take sample form ADC and send it over softserial . load the following code to arduino.
int id=99; #include <SoftwareSerial.h> SoftwareSerial sw(2, 3); // RX, TX void setup() { Serial.begin(115200); Serial.println("Interfacfing arduino with nodemcu"); sw.begin(115200); } void loop() { Serial.println("Sending data to nodemcu"); int adc=analogRead(A0); Serial.print("{\"sensorid\":"); Serial.print(id);//sensor id Serial.print(","); Serial.print("\"adcValue\":"); Serial.print(adc);//offset Serial.print("}"); Serial.println(); sw.print("{\"sensorid\":"); sw.print(id);//sensor id sw.print(","); sw.print("\"adcValue\":"); sw.print(adc);//offset sw.print("}"); sw.println(); delay(5000); }

NodeMCU code:
On nodemcu you need to load 4 files, all are available on github.
init.lua
config.lua (edit your wifi,MQTT broker details here)
setup.lua
app.lua
Load all these three files to nodemcu. To test the setup, send a message from console , it should appear in your MQTT channel.

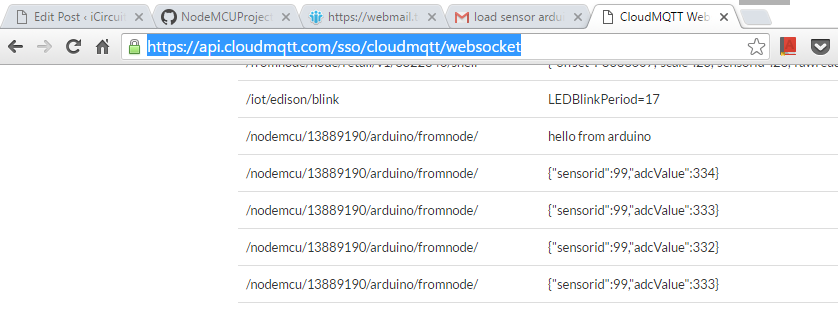
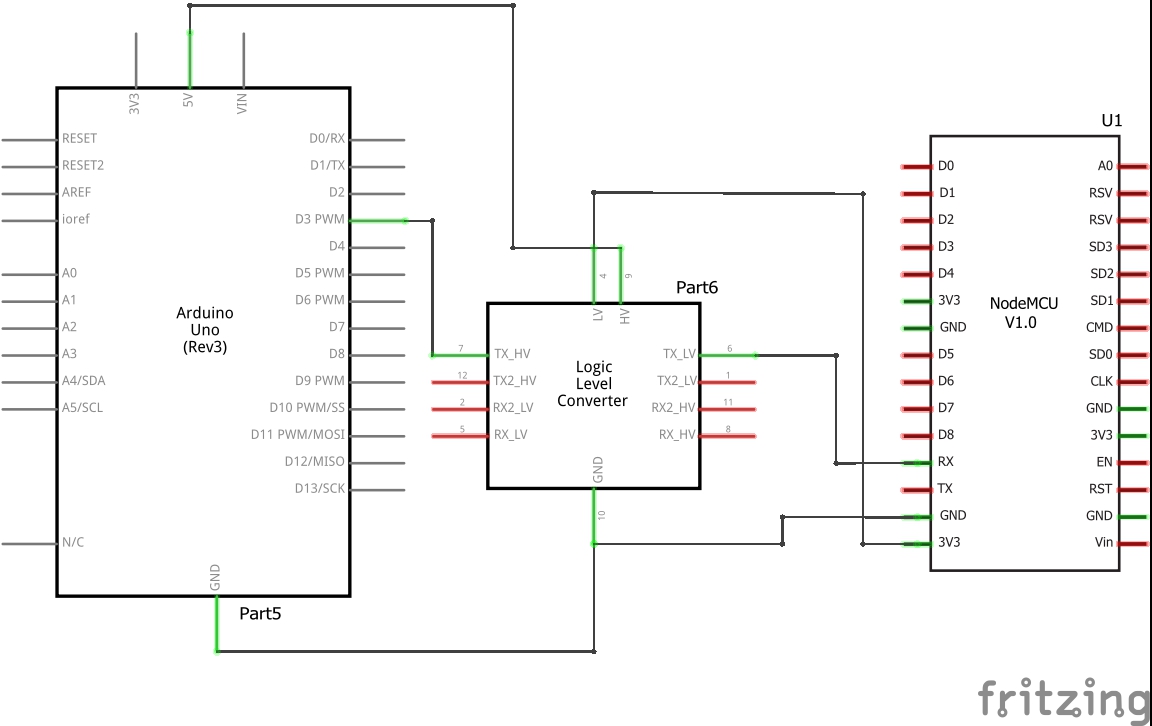
Once you verified that the nodemcu is able to connect to broker and send messages, you can connect Arduino to nodemcu. First you should close the serial port connection to nodemcu , then Connect Arduino SoftSerial Tx to NodeMCU Rx (through level shifter).
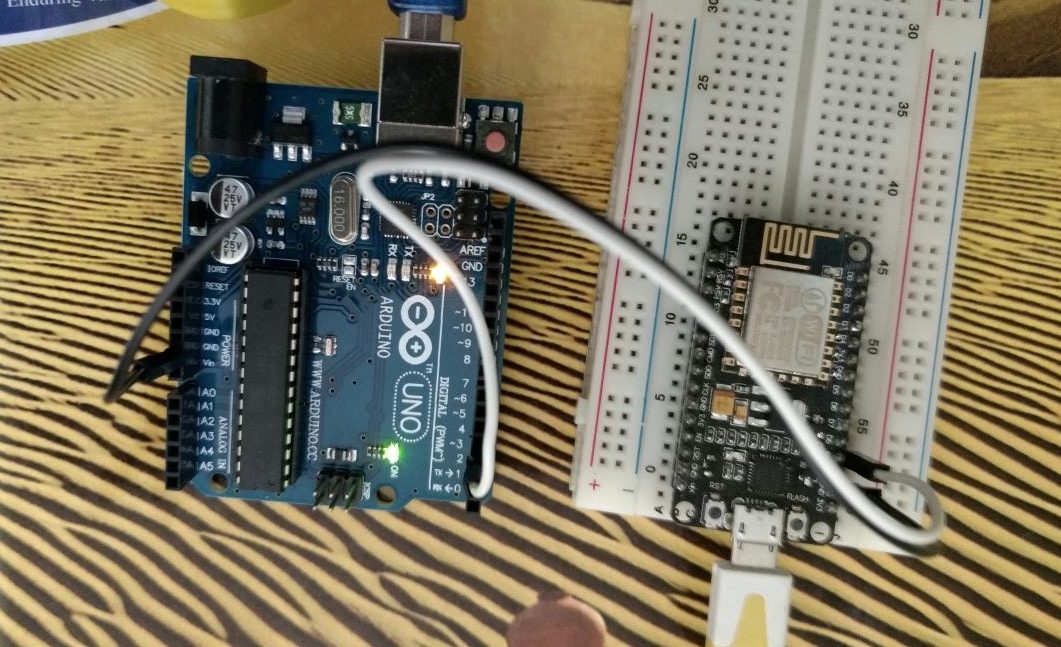
Note : This post uses Lua for programming NodeMCU, if you want to use Arduino IDE, checkout this post
If I am using my Arduino IDE to programme my Node MCU as well, can I use any pin (D0-D8) to be my Rx-Tx “mySerial” using SoftwareSerial like the ones we use in Arduino
I haven’t tried that, softserial requires interrupt support on IOs, it might work
looks like, it should work
http://esp8266.github.io/Arduino/versions/2.3.0/doc/libraries.html#softwareserial
can we send mpu6050 motion sensor readings to webpage using arduino ide?
Hi,
you can’t send to data to webpage from arduino IDE, you need to have some sort server which will read from serial port and sent to webpage using websockets or mqtt
In the text you have written that “Connect Arduino SoftSerial Tx to NodeMCU Rx (through level shifter)”. But In the wiring diagram given in the article Rx of arduino is connected to Rx of nodemcu. Also no level shifter is shown in the pic. Can you explain? i am a beginner in this field.
Jeevan
Hi, seems the picture is not clear , Rx of nodemcu is connected to Tx of softserial, otherwise it won’t work. I haven’t used level shifter (in some cases it might damage the nodemcu,thats why i suggested to use a level shifter). In most cases it works without any level shifter.
Instead of level shifter can I use voltage regulator IC LD33v(Its used to bring 5v to 3.3v )
Sir i withdraw the first part of my question ( reg the Tx&Rx pins). Could you pl help me with the level shifter part of my question? Thanks.
http://icircuit.net/use-level-shifters/1719
I want to send data from my phone to arduino using nodemcu(as nodemcu has wifi facility), I should be able to send data to my arduino from any place(over the internet).I am not able to figure out how to do this. If you know please help me out with this. Thanking you in advance.
yes you can send data/commands from mobile to arduino.
check out this blogs
http://icircuit.net/remote-pwm-nodemcu-and-mqtt/897
http://icircuit.net/nodemcu-based-intruder-detector/1010
i want to connect arduino uno motor driver shield in to nodemcu how can i do that how to connect pin.please help me
can you let me know the motor driver details
Can i connect a NodeMCU and HC SR04 to arduino uno and upload sensor values to thingspeak server ? can u please give an idea on the pin connections ?
yes, you can connect. you can hook-up the sr04 to arduino take the readings. These readings can be feed to the nodemcu which will publish it to thingspeak server
Hello sir,
How can i upload init.lua ,config.lua, setup.lua, app.lua programs to ESP 8266 -12E nodemcu mudules. plz help me.
Refer following blog to see how to load code to nodemcu http://icircuit.net/setting-up-node-mcu/743
I am collecting data on arduino through an analog pin and I want to transfer the data to my mobile using nodemcu. How can I do that? please help.
Hi,
By following this blog you can get the data to mqtt broker, you can use a mqtt lib in your android app to receive data from the mqtt broker
Hi Sankar,
Excellent article. I really love the way how easily you articulate difficult topics.
I wanted your help, as got stuck in uploading the 4 Lua files in this article. I am getting different errors and basically Wifi is not getting connected. I tried with different NodeMCU firmware and get different results every time. Can you please advise what NodeMCU build are you using? Thanks Regards Tushar
Hi Banwett,
Thanks, I am using this tool https://nodemcu-build.com/
please share to me Library SoftWareSerial for ESP32 DEV board,
Thanks
Hello. you are grate. please can you answer? i have set couple of sensors and modules on arduino. now i want to control and see tham on blynk app. can i do it with nodemcu ? thank u
Hi,I haven’t worked with blynk yet,But I feel it should be possible
i am getting error like connecting to broker and nothing happens after that can anyone help me out
Hi,could you provide more details about the problem
Can you explain how to load that 4 files in nodemcu
Hi, You can load lua files to NodeMCU by clicking on Save&Run button in the IDE
Sir please clearly mention the hardware connection…. of arduino and nodemcu during serial transmission of data
I’d like to know if there is any way to connect NodeMcu to arduino using I2C , Node mcu will act like a master and arduino as a slave, because I want to plug relay to my arduino since I dont have enough pins on NodeMcu and control that relay using NodeMcu,
thanks for any indication
Hi, You can get additional IOs for NodeMCU by using shift registers.
you can refer this post
http://icircuit.net/extending-nodemcu-output-pins-shift-registers/1739
¿Porqué id=99? gracias.
Hi, the number has no significance, it can be anything
Hi, Thank you for a good post. I am doing project that need something like this. However, I do not have logic gate converter. Do I need logic gate converter to connect arduino and nodemcu?
it is advisable to use level converter, seems most of the nodemcu tolerate 5v on signal line
not getting proper values.. i have selected node mcu board
Hello,
Thats a very nice article, i want to send two sensor values from my ardiuno to node mcu..so can u tell me the hardware connections is same as u have done in this article or it has to be changed?
it will be save, connect your sensors to analog pins, if they provide analog out
Hi, thanks for your explanation. Is it possible for me to send data from ultrasonic sensor (that connected to Uno board) to NodeMcu then send to server? I tried so many code but it doesnt work 🙁 Could you please help me
I want to send data of current sensor from arduino to nodemcu. Can you guide me on how to do this?
Hello Sir,
thanks a lot for this post. i have just started with arduino & nodeMCU. i want to show the temp, humidity and distance on blynk. my DHT11 & HC-SR04 connected to arduino uno (pin2, 3 & 4) , but my blynk is communicating to nodeMCU. i want to communicate the arduino & nodeMCU together, so that i can read the value from arduino uno to nodeMCU. i am using Arduino IDE software for programming of both. please post nodeMCU code & arduino code.
Hi, if you have the solution for this, can you share because I have got problem to connect arduino with nodemcu too
Hello Sir,
thanks a lot for this post
i want connect nodemcu esp8266 to arduino mega 2560 in order to transfer information from another nodemcu to this nodemcu and then this nodemcu transfers to arduino . in my project arduino is master and two nodemcu are slave.
one nodemcu connect to sensors far from another nodemcu that connected to arduino
can i use your wiring in my project ?
tx connect to rx and rx connect to tx
please guide me
best regards
sir, i have this question hope you have the answer of. I am doing this led matrix project project where user can make any text to appear on the scrolling led dot matrix. I am having trouble setting up the serial connection between my arduino with the nodemcu. i want the nodemcu to recieve the data(that the user would enter on a web page) and then it would be trasmitted to the arduino so whatever message the user wants is displayed on the matrix display. I’d be so happy if you can help me with this
Hi,
Rather then connecting the 5v Arduino board to 3.3v nodemcu for power supply, can I directly power the nodemcu with my laptop through USB
Yes, you can power nodemcu from laptop, don’t forget common the gnd
Hello, I’m currently doing a project that uses an Arduino nano, and an esp32. My question is why don’t you use the TX and RX on the Arduino? If you want to communicate by receiving or transmitting data, other schematics show that you have to have to use the TX and RX. I don’t know how I should connect the 2 devices.
Hi, you can use the hardware Tx,Rx pins. I didn’t use because I wanted to use the serial monitor as well.
invalid characters appearing on my terminal not sure why 🙁
solved , was due to different baud rate , also can cause by different voltage if you are using esp866 is its pins are 3v
I am having 5 sensors connected to arduino mega. I need to send data to thingspeak using nodemcu esp8266. How to connect arduino mega and esp8266?
what is the interfacing node mcu to thing cloud board